STACK Programming in C
STACK Programming in C Language-
Stack Data Structure
Stack is a linear data structure which follows a particular order in which the operations are performed. The order may be LIFO(Last In First Out) or FILO(First In Last Out).
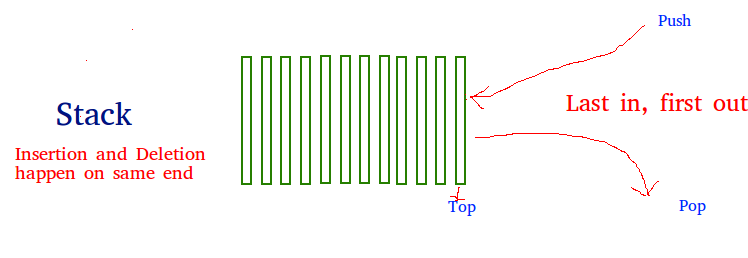
There are many real-life examples of a stack. Consider an example of plates stacked over one another in the canteen. The plate which is at the top is the first one to be removed, i.e. the plate which has been placed at the bottommost position remains in the stack for the longest period of time. So, it can be simply seen to follow LIFO(Last In First Out)/FILO(First In Last Out) order.
problem statement:perform the following operations -1. PUSH,
2.POP
3.PEEP
4.DISPLAY
C-CODE:
#include
#include
#include
#define MAX 5//MAX is an inbuilt macro in C
int top=-1;
int stack[MAX];
void push();
void pop();
void peep();
void display();
void main()
{
char ch;
while(1)
{
printf("\n 1:push,\n2:pop,\n3:peep\n4:display\n0:Exit\n");
printf("enter your choice\n");
scanf("%d",&ch);
switch(ch)
{
case 1:
{
push();
break;
}
case 2:
{
pop();
break;
} case 3:
{
peep();
break;
}
case 4:
{
display();
break;
}
case 0:
{
exit(0);
}
default :
{
printf("wrong choice");
break;
}
}
getch();
}
}
void push()//entering of element
{
int x;
if(top==MAX-1)
{
printf("overflow\n");
}
else
{
printf("enter the element to be inserted\n");
scanf("%d",&x); top++;
stack[top]=x;
}
return;
}
void pop()//deletion of element
{
int item;
if(top==-1)
printf("underflow");
else
{
item=stack[top];
top--;
printf("the deleted element is %d\n",item);
}
return;
}
#include
#include
#define MAX 5//MAX is an inbuilt macro in C
int top=-1;
int stack[MAX];
void push();
void pop();
void peep();
void display();
void main()
{
char ch;
while(1)
{
printf("\n 1:push,\n2:pop,\n3:peep\n4:display\n0:Exit\n");
printf("enter your choice\n");
scanf("%d",&ch);
switch(ch)
{
case 1:
{
push();
break;
}
case 2:
{
pop();
break;
} case 3:
{
peep();
break;
}
case 4:
{
display();
break;
}
case 0:
{
exit(0);
}
default :
{
printf("wrong choice");
break;
}
}
getch();
}
}
void push()//entering of element
{
int x;
if(top==MAX-1)
{
printf("overflow\n");
}
else
{
printf("enter the element to be inserted\n");
scanf("%d",&x); top++;
stack[top]=x;
}
return;
}
void pop()//deletion of element
{
int item;
if(top==-1)
printf("underflow");
else
{
item=stack[top];
top--;
printf("the deleted element is %d\n",item);
}
return;
}
void peep()//will display the topmost element of stack
{printf("the top most element is %d",stack[top]);
return;
}
void display()
{
int i;
printf("the stack is\n");
for(i=top;i>=0;i--)
{
printf("%d\n",stack[i]);
}
return;
}
THE PROGRAM HAS BEEN COMPLETED. DO IT BY YOURSELF AFTER UNDERSTANDING.
Comments
Post a Comment